Table of Contents
In the modern digital age, uninterrupted communication is paramount for businesses striving to connect with their customers effectively.
WhatsApp, with its extensive global reach, has become a preferred channel for direct messaging. Integrating WhatsApp messaging into your applications can enhance engagement, automate notifications, and provide instant customer support. Leveraging the Meta Developer API, businesses can harness the power of WhatsApp programmatically using Node.js, a popular JavaScript runtime.
This guide is designed to walk you through the process of sending WhatsApp messages using Node.js, from setting up your development environment to executing your first message. Whether you're building a customer support bot, a notification system, or simply experimenting with WhatsApp’s capabilities, this tutorial will provide a comprehensive, step-by-step approach to achieving your goals.
Integrating WhatsApp Messaging Into Your Node.js Application
- Real-Time User Engagement:
Achieve instant communication with your audience, fostering more immediate and meaningful interactions.
- Automate Routine Tasks:
Streamline processes like notifications, reminders, and customer support, reducing manual workload and improving efficiency.
- Rich Messaging Capabilities:
Utilize diverse media formats, including text, images, and videos, to create more engaging and dynamic user experiences.
- Global Accessibility:
Reach out to a broad audience across various regions, leveraging WhatsApp’s extensive global user base.
- Customizable Interaction:
Design personalized messaging solutions tailored to the specific needs and preferences of your users.
-
Streamlined API Integration:
Take advantage of Meta’s comprehensive API to integrate WhatsApp messaging seamlessly into your Node.js app.
-
Stay Updated with New Features:
Benefit from ongoing updates and enhancements to the WhatsApp API, ensuring you leverage the latest advancements.
- Enhanced User Retention:
Increase customer satisfaction and loyalty through timely and relevant communication.
Prerequisites
Before diving into the code, ensure you have the following:
- Meta Developer Account: You need access to the Meta for Developers portal, where you'll set up your WhatsApp API account.
- Node.js Installed: Ensure Node.js is installed on your machine. You can download it from Node.js.
- WhatsApp Business Account: Your WhatsApp Business API setup should be completed, with an active phone number linked to your account.
- Access Token: An access token from the Meta Developer Portal to authenticate API requests.
Step 1: Setting Up Your Node.js Project
Start by creating a new Node.js project. In your terminal, run:
mkdir whatsapp-nodejs
cd whatsapp-nodejs
npm init -y
This creates a new directory for your project and initializes it with a package.json file.
Step 2: Install Required Packages
You need a package to make HTTP requests. We'll use axios for this purpose:
npm install axios
Step 3: Writing the Code
Create a new file index.js in the project directory. This file will contain the code to send a WhatsApp message.
Here’s the step-by-step code:
-
Import Required Modules:
const axios = require('axios');
The axios module is imported to handle HTTP requests.
-
Set Up Your Configuration:
const token = 'YOUR_ACCESS_TOKEN';
const phoneNumberId = 'YOUR_PHONE_NUMBER_ID';
const recipient phone number = 'RECIPIENT_PHONE_NUMBER';
Replace YOUR_ACCESS_TOKEN with your Meta API access token, YOUR_PHONE_NUMBER_ID with your WhatsApp Business Account phone number ID, and RECIPIENT_PHONE_NUMBER with the phone number you want to send the message to.
- Construct the API Endpoint:
const apiUrl = `https://graph.facebook.com/v16.0/${phoneNumberId}/messages`;
This URL is where you’ll send the HTTP POST request to send a message. The URL includes the version of the API (v16.0) and your specific phone number ID.
-
Create the Message Payload:
const data = {
messaging_product: "whatsapp",
to: recipientPhoneNumber,
type: "text",
text: {
body: "Hello, this is a test message from my Node.js app!"
}
};
This payload specifies the type of messaging product (WhatsApp), the recipient's phone number, the type of message (text), and the message body.
- Send the Message:
axios.post(apiUrl, data, {
headers: {
Authorization: `Bearer ${token}`,
'Content-Type': 'application/json'
}
})
.then(response => {
console.log('Message sent successfully:', response.data);
})
.catch(error => {
console.error('Error sending message:', error.response.data);
});
Here, you’re using axios.post() to send the message. The Authorization header includes your access token, and the Content-Type header specifies that the request body is JSON. The response from the API is logged to the console, and any errors are handled and displayed.
Step 4: Running the Script
With everything set up, run your script using Node.js:
node index.js
If everything is configured correctly, you should see a message indicating that the message was sent successfully.
Step 5: Areas to Be Careful About
- Access Token: Ensure your access token is kept secure and refreshed as needed, as tokens may expire.
- Recipient Phone Number Format: Use the correct format for phone numbers, including the country code but without the + sign (e.g., 1234567890 for a US number).
- API Versioning: Always use the latest stable version of the API to avoid deprecated features.
- Error Handling: Implement comprehensive error handling in production environments to manage issues such as network errors or invalid responses.
Enhance Your Communication with WhatsApp Integration!
Integrate WhatsApp messaging into your Node.js app with our step-by-step guide.
Conclusion
By following this guide, you have successfully set up a Node.js environment to send WhatsApp messages using the Meta Developer API. This foundational knowledge can be extended to support various types of messages, including multimedia content like images, videos, and documents.
As you develop your application further, always refer to the official Meta documentation for updates and best practices. Integrating WhatsApp messaging into your applications can significantly enhance communication, improve customer engagement, and streamline operational workflows.
Table of Contents
In the modern digital age, uninterrupted communication is paramount for businesses striving to connect with their customers effectively.
WhatsApp, with its extensive global reach, has become a preferred channel for direct messaging. Integrating WhatsApp messaging into your applications can enhance engagement, automate notifications, and provide instant customer support. Leveraging the Meta Developer API, businesses can harness the power of WhatsApp programmatically using Node.js, a popular JavaScript runtime.
This guide is designed to walk you through the process of sending WhatsApp messages using Node.js, from setting up your development environment to executing your first message. Whether you're building a customer support bot, a notification system, or simply experimenting with WhatsApp’s capabilities, this tutorial will provide a comprehensive, step-by-step approach to achieving your goals.
Integrating WhatsApp Messaging Into Your Node.js Application
- Real-Time User Engagement:
Achieve instant communication with your audience, fostering more immediate and meaningful interactions.
- Automate Routine Tasks:
Streamline processes like notifications, reminders, and customer support, reducing manual workload and improving efficiency.
- Rich Messaging Capabilities:
Utilize diverse media formats, including text, images, and videos, to create more engaging and dynamic user experiences.
- Global Accessibility:
Reach out to a broad audience across various regions, leveraging WhatsApp’s extensive global user base.
- Customizable Interaction:
Design personalized messaging solutions tailored to the specific needs and preferences of your users.
-
Streamlined API Integration:
Take advantage of Meta’s comprehensive API to integrate WhatsApp messaging seamlessly into your Node.js app.
-
Stay Updated with New Features:
Benefit from ongoing updates and enhancements to the WhatsApp API, ensuring you leverage the latest advancements.
- Enhanced User Retention:
Increase customer satisfaction and loyalty through timely and relevant communication.
Prerequisites
Before diving into the code, ensure you have the following:
- Meta Developer Account: You need access to the Meta for Developers portal, where you'll set up your WhatsApp API account.
- Node.js Installed: Ensure Node.js is installed on your machine. You can download it from Node.js.
- WhatsApp Business Account: Your WhatsApp Business API setup should be completed, with an active phone number linked to your account.
- Access Token: An access token from the Meta Developer Portal to authenticate API requests.
Step 1: Setting Up Your Node.js Project
Start by creating a new Node.js project. In your terminal, run:
mkdir whatsapp-nodejs
cd whatsapp-nodejs
npm init -y
This creates a new directory for your project and initializes it with a package.json file.
Step 2: Install Required Packages
You need a package to make HTTP requests. We'll use axios for this purpose:
npm install axios
Step 3: Writing the Code
Create a new file index.js in the project directory. This file will contain the code to send a WhatsApp message.
Here’s the step-by-step code:
-
Import Required Modules:
const axios = require('axios');
The axios module is imported to handle HTTP requests.
-
Set Up Your Configuration:
const token = 'YOUR_ACCESS_TOKEN';
const phoneNumberId = 'YOUR_PHONE_NUMBER_ID';
const recipient phone number = 'RECIPIENT_PHONE_NUMBER';
Replace YOUR_ACCESS_TOKEN with your Meta API access token, YOUR_PHONE_NUMBER_ID with your WhatsApp Business Account phone number ID, and RECIPIENT_PHONE_NUMBER with the phone number you want to send the message to.
- Construct the API Endpoint:
const apiUrl = `https://graph.facebook.com/v16.0/${phoneNumberId}/messages`;
This URL is where you’ll send the HTTP POST request to send a message. The URL includes the version of the API (v16.0) and your specific phone number ID.
-
Create the Message Payload:
const data = {
messaging_product: "whatsapp",
to: recipientPhoneNumber,
type: "text",
text: {
body: "Hello, this is a test message from my Node.js app!"
}
};
This payload specifies the type of messaging product (WhatsApp), the recipient's phone number, the type of message (text), and the message body.
- Send the Message:
axios.post(apiUrl, data, {
headers: {
Authorization: `Bearer ${token}`,
'Content-Type': 'application/json'
}
})
.then(response => {
console.log('Message sent successfully:', response.data);
})
.catch(error => {
console.error('Error sending message:', error.response.data);
});
Here, you’re using axios.post() to send the message. The Authorization header includes your access token, and the Content-Type header specifies that the request body is JSON. The response from the API is logged to the console, and any errors are handled and displayed.
Step 4: Running the Script
With everything set up, run your script using Node.js:
node index.js
If everything is configured correctly, you should see a message indicating that the message was sent successfully.
Step 5: Areas to Be Careful About
- Access Token: Ensure your access token is kept secure and refreshed as needed, as tokens may expire.
- Recipient Phone Number Format: Use the correct format for phone numbers, including the country code but without the + sign (e.g., 1234567890 for a US number).
- API Versioning: Always use the latest stable version of the API to avoid deprecated features.
- Error Handling: Implement comprehensive error handling in production environments to manage issues such as network errors or invalid responses.
Enhance Your Communication with WhatsApp Integration!
Integrate WhatsApp messaging into your Node.js app with our step-by-step guide.
Conclusion
By following this guide, you have successfully set up a Node.js environment to send WhatsApp messages using the Meta Developer API. This foundational knowledge can be extended to support various types of messages, including multimedia content like images, videos, and documents.
As you develop your application further, always refer to the official Meta documentation for updates and best practices. Integrating WhatsApp messaging into your applications can significantly enhance communication, improve customer engagement, and streamline operational workflows.
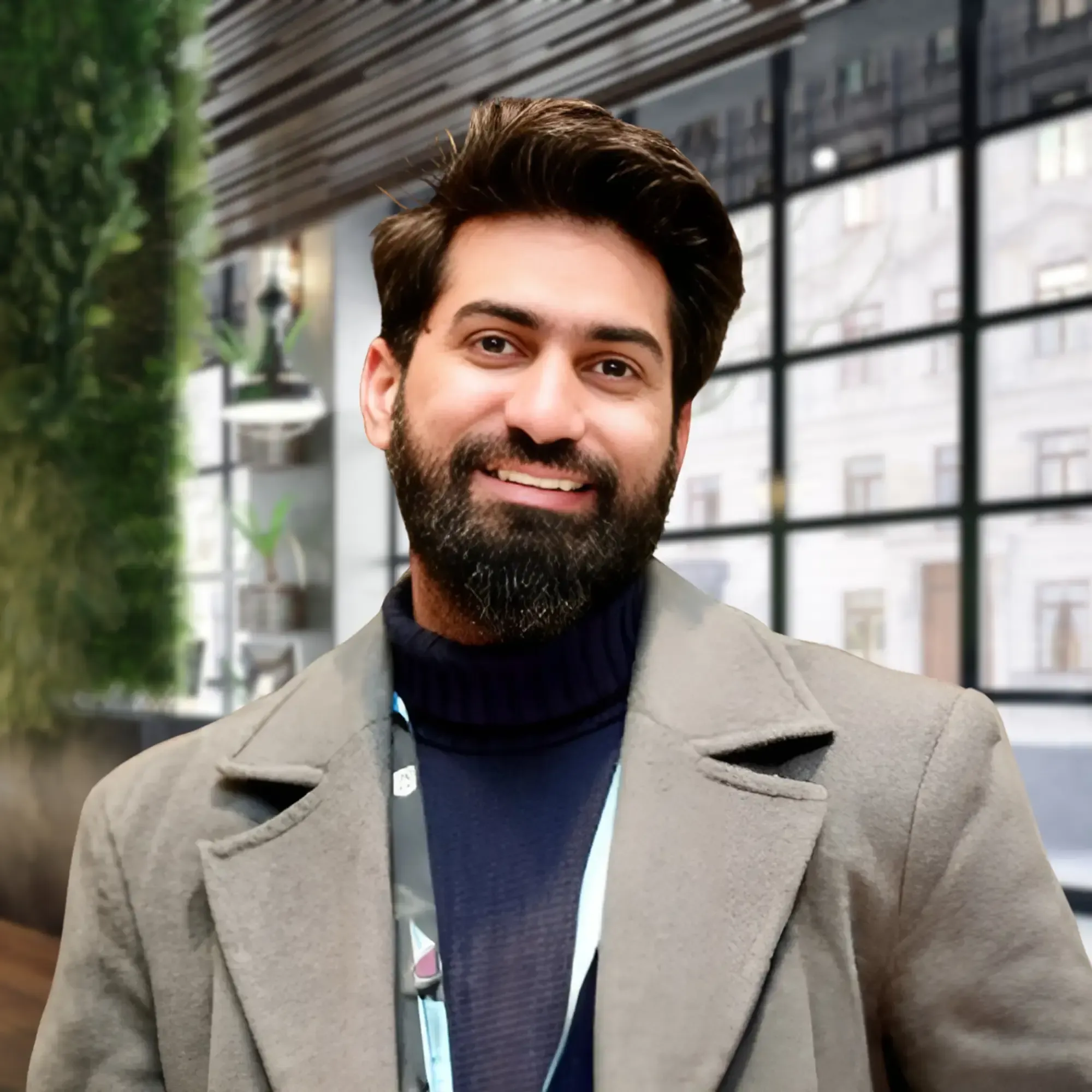
Written by :
Furqan Aziz
Furqan Aziz is CEO & Founder of InvoTeams. He is a tech enthusiast by heart with 10+ years of development experience & occasionally writes about technology here.