Table of Contents
- Introduction
- After Reading This Blog Post, You’ll Know:
- Step 1: Set Up Your Development Environment
- Step 2: Initialize Your React Native Project
- Step 3: Set Up Firebase
- Step 4: Implement User Authentication
- Step 5: Build the Chat Interface
- Step 6: Add Real-time Messaging
- Step 7: Implement Media Sharing
- Step 8: Test Your Application
- Step 9: Deploy and Scale
- Get Started with Your Chat App!
- Conclusion
Introduction
Building a chat application akin to WhatsApp is an exciting venture that combines creativity with technology. This guide will guide you through the essential steps to create a robust, real-time chat application from scratch.
Using modern tools and technologies such as Node.js, React Native, and Firebase, you'll learn how to craft a dynamic, cross-platform app that allows users to chat seamlessly, just like they would in their favorite messaging apps.
Whether you're a seasoned developer or just getting started, this guide will provide you with the knowledge and tools to bring your chat app idea to life.
After Reading This Blog Post, You’ll Know:
- Project Initialization: Steps to create and initialize a React Native project with Expo.
- Firebase Integration: How to connect Firebase for authentication and real-time database management.
- User Authentication: Techniques to implement secure login and registration features.
- Chat Interface Design: Designing an intuitive chat interface for seamless communication.
- Real-Time Messaging: Using Firebase to ensure instant message delivery and updates.
- Media Sharing: Adding functionality for users to share images and media.
- Testing and Deployment: Testing your app on various devices and deploying it for real-world use.
Step 1: Set Up Your Development Environment
Before starting the development, ensure you have the necessary tools and software installed:
- Node.js: For backend development.
- React Native: For building cross-platform mobile applications.
- Firebase: For real-time database and authentication.
- Expo CLI: For managing the React Native app.
Install Node.js and then use NPM (Node Package Manager) to install React Native and Expo CLI:
npm install -g expo-cli
Step 2: Initialize Your React Native Project
Create a new React Native project using Expo:
expo init ChatApp
cd ChatApp
Choose a template and follow the prompts to set up your project. Once initialized, you can start the development server:
expo start
Step 3: Set Up Firebase
- Create a Firebase Project: Go to the Firebase Console and create a new project.
- Enable Authentication: Go to the Authentication section in the Firebase Console and enable Email/Password authentication.
- Create a Realtime Database: Go to the Database section and create a new Realtime Database. Choose the test mode to allow read/write access during development.
- Install Firebase SDK: Add Firebase to your React Native project.
npm install firebase
-
Configure Firebase in Your App: In your project, create a firebase.js file to initialize Firebase:
// firebase.js
import firebase from 'firebase/app';
import 'firebase/auth';
import 'firebase/database';
const firebaseConfig = {
apiKey: "YOUR_API_KEY",
authDomain: "YOUR_PROJECT_ID.firebaseapp.com",
databaseURL: "https://YOUR_PROJECT_ID.firebaseio.com",
projectId: "YOUR_PROJECT_ID",
storageBucket: "YOUR_PROJECT_ID.appspot.com",
messagingSenderId: "YOUR_SENDER_ID",
appId: "YOUR_APP_ID"
};
if (!firebase.apps.length) {
firebase.initializeApp(firebaseConfig);
}
export { firebase };
Step 4: Implement User Authentication
Create a simple login and registration screen using Firebase Authentication. You can use React Native components and hooks for handling state and input.
// SignInScreen.js
import React, { useState } from 'react';
import { View, TextInput, Button, Text } from 'react-native';
import { firebase } from './firebase';
export default function SignInScreen() {
const [email, setEmail] = useState('');
const [password, setPassword] = useState('');
const signIn = async () => {
try {
await firebase.auth().signInWithEmailAndPassword(email, password);
// Navigate to chat screen
} catch (error) {
console.error(error);
}
};
return (
<View>
<TextInput placeholder="Email" value={email} onChangeText={setEmail} />
<TextInput
placeholder="Password"
value={password}
onChangeText={setPassword}
secureTextEntry
/>
<Button title="Sign In" onPress={signIn} />
</View>
);
}
Step 5: Build the Chat Interface
Use React Native components to build a chat interface. A simple chat screen includes a message list and an input field to send messages.
// ChatScreen.js
import React, { useState, useEffect } from 'react';
import { View, TextInput, Button, FlatList, Text } from 'react-native';
import { firebase } from './firebase';
export default function C
hatScreen() {
const [message, setMessage] = useState('');
const [messages, setMessages] = useState([]);
useEffect(() => {
const messageRef = firebase.database().ref('messages');
messageRef.on('value', (snapshot) => {
const data = snapshot.val();
const parsedMessages = Object.values(data || {});
setMessages(parsedMessages);
});
}, []);
const sendMessage = () => {
const messageRef = firebase.database().ref('messages');
const newMessage = {
text: message,
timestamp: firebase.database.ServerValue.TIMESTAMP,
};
messageRef.push(newMessage);
setMessage('');
};
return (
<View style={{ flex: 1 }}>
<FlatList
data={messages}
renderItem={({ item }) => <Text>{item.text}</Text>}
keyExtractor={(item, index) => index.toString()}
/>
<TextInput
placeholder="Type a message"
value={message}
onChangeText={setMessage}
/>
<Button title="Send" onPress={sendMessage} />
</View>
);
}
Step 6: Add Real-time Messaging
Use Firebase's real-time capabilities to update the chat interface instantly when new messages are sent. The useEffect hook listens for changes in the messages’ reference and updates the state accordingly.
Step 7: Implement Media Sharing
To implement media sharing, you can use React Native libraries like react-native-image-picker for selecting images and Firebase Storage for uploading them.
- Install the Image Picker:
npm install react-native-image-picker
-
Upload Images to Firebase Storage:
import { launchImageLibrary } from 'react-native-image-picker';
import 'firebase/storage';
const uploadImage = async () => {
const result = await launchImageLibrary({ mediaType: 'photo' });
if (result.assets) {
const { uri } = result.assets[0];
const response = await fetch(uri);
const blob = await response.blob();
const storageRef = firebase.storage().ref(`images/${Date.now()}`);
await storageRef.put(blob);
const downloadURL = await storageRef.getDownloadURL();
sendMessage(downloadURL, 'image');
}
};
const sendMessage = (content, type = 'text') => {
const messageRef = firebase.database().ref('messages');
const newMessage = {
content,
type,
timestamp: firebase.database.ServerValue.TIMESTAMP,
};
messageRef.push(newMessage);
setMessage('');
};
Step 8: Test Your Application
Run your application using Expo to test it on both Android and iOS devices:
expo start
Use the Expo Go app on your mobile device to scan the QR code and test the functionality.
Step 9: Deploy and Scale
Once development and testing are completed, consider deploying your backend to a cloud service like Google Cloud or AWS for scalability. Optimize your application for performance and security.
Get Started with Your Chat App!
Explore our step-by-step guide to create a chat application like WhatsApp. Dive into real-time messaging, user authentication, and more!
Conclusion
Are you considering to develop your web app, that highly resembles whatsapp features? Remember that developing a chat application like WhatsApp is both a challenging and rewarding project. By following this guide, you've laid the groundwork for a real-time messaging platform with essential features like user authentication, instant messaging, and media sharing.
As you continue to develop and refine your app, remember that the possibilities are endless, consider exploring advanced features like voice and video calls or adding robust encryption for enhanced security.
With your new skills and a solid foundation, you're well on your way to building a compelling chat experience that users will love. Happy coding!
Table of Contents
- Introduction
- After Reading This Blog Post, You’ll Know:
- Step 1: Set Up Your Development Environment
- Step 2: Initialize Your React Native Project
- Step 3: Set Up Firebase
- Step 4: Implement User Authentication
- Step 5: Build the Chat Interface
- Step 6: Add Real-time Messaging
- Step 7: Implement Media Sharing
- Step 8: Test Your Application
- Step 9: Deploy and Scale
- Get Started with Your Chat App!
- Conclusion
Introduction
Building a chat application akin to WhatsApp is an exciting venture that combines creativity with technology. This guide will guide you through the essential steps to create a robust, real-time chat application from scratch.
Using modern tools and technologies such as Node.js, React Native, and Firebase, you'll learn how to craft a dynamic, cross-platform app that allows users to chat seamlessly, just like they would in their favorite messaging apps.
Whether you're a seasoned developer or just getting started, this guide will provide you with the knowledge and tools to bring your chat app idea to life.
After Reading This Blog Post, You’ll Know:
- Project Initialization: Steps to create and initialize a React Native project with Expo.
- Firebase Integration: How to connect Firebase for authentication and real-time database management.
- User Authentication: Techniques to implement secure login and registration features.
- Chat Interface Design: Designing an intuitive chat interface for seamless communication.
- Real-Time Messaging: Using Firebase to ensure instant message delivery and updates.
- Media Sharing: Adding functionality for users to share images and media.
- Testing and Deployment: Testing your app on various devices and deploying it for real-world use.
Step 1: Set Up Your Development Environment
Before starting the development, ensure you have the necessary tools and software installed:
- Node.js: For backend development.
- React Native: For building cross-platform mobile applications.
- Firebase: For real-time database and authentication.
- Expo CLI: For managing the React Native app.
Install Node.js and then use NPM (Node Package Manager) to install React Native and Expo CLI:
npm install -g expo-cli
Step 2: Initialize Your React Native Project
Create a new React Native project using Expo:
expo init ChatApp
cd ChatApp
Choose a template and follow the prompts to set up your project. Once initialized, you can start the development server:
expo start
Step 3: Set Up Firebase
- Create a Firebase Project: Go to the Firebase Console and create a new project.
- Enable Authentication: Go to the Authentication section in the Firebase Console and enable Email/Password authentication.
- Create a Realtime Database: Go to the Database section and create a new Realtime Database. Choose the test mode to allow read/write access during development.
- Install Firebase SDK: Add Firebase to your React Native project.
npm install firebase
-
Configure Firebase in Your App: In your project, create a firebase.js file to initialize Firebase:
// firebase.js
import firebase from 'firebase/app';
import 'firebase/auth';
import 'firebase/database';
const firebaseConfig = {
apiKey: "YOUR_API_KEY",
authDomain: "YOUR_PROJECT_ID.firebaseapp.com",
databaseURL: "https://YOUR_PROJECT_ID.firebaseio.com",
projectId: "YOUR_PROJECT_ID",
storageBucket: "YOUR_PROJECT_ID.appspot.com",
messagingSenderId: "YOUR_SENDER_ID",
appId: "YOUR_APP_ID"
};
if (!firebase.apps.length) {
firebase.initializeApp(firebaseConfig);
}
export { firebase };
Step 4: Implement User Authentication
Create a simple login and registration screen using Firebase Authentication. You can use React Native components and hooks for handling state and input.
// SignInScreen.js
import React, { useState } from 'react';
import { View, TextInput, Button, Text } from 'react-native';
import { firebase } from './firebase';
export default function SignInScreen() {
const [email, setEmail] = useState('');
const [password, setPassword] = useState('');
const signIn = async () => {
try {
await firebase.auth().signInWithEmailAndPassword(email, password);
// Navigate to chat screen
} catch (error) {
console.error(error);
}
};
return (
<View>
<TextInput placeholder="Email" value={email} onChangeText={setEmail} />
<TextInput
placeholder="Password"
value={password}
onChangeText={setPassword}
secureTextEntry
/>
<Button title="Sign In" onPress={signIn} />
</View>
);
}
Step 5: Build the Chat Interface
Use React Native components to build a chat interface. A simple chat screen includes a message list and an input field to send messages.
// ChatScreen.js
import React, { useState, useEffect } from 'react';
import { View, TextInput, Button, FlatList, Text } from 'react-native';
import { firebase } from './firebase';
export default function C
hatScreen() {
const [message, setMessage] = useState('');
const [messages, setMessages] = useState([]);
useEffect(() => {
const messageRef = firebase.database().ref('messages');
messageRef.on('value', (snapshot) => {
const data = snapshot.val();
const parsedMessages = Object.values(data || {});
setMessages(parsedMessages);
});
}, []);
const sendMessage = () => {
const messageRef = firebase.database().ref('messages');
const newMessage = {
text: message,
timestamp: firebase.database.ServerValue.TIMESTAMP,
};
messageRef.push(newMessage);
setMessage('');
};
return (
<View style={{ flex: 1 }}>
<FlatList
data={messages}
renderItem={({ item }) => <Text>{item.text}</Text>}
keyExtractor={(item, index) => index.toString()}
/>
<TextInput
placeholder="Type a message"
value={message}
onChangeText={setMessage}
/>
<Button title="Send" onPress={sendMessage} />
</View>
);
}
Step 6: Add Real-time Messaging
Use Firebase's real-time capabilities to update the chat interface instantly when new messages are sent. The useEffect hook listens for changes in the messages’ reference and updates the state accordingly.
Step 7: Implement Media Sharing
To implement media sharing, you can use React Native libraries like react-native-image-picker for selecting images and Firebase Storage for uploading them.
- Install the Image Picker:
npm install react-native-image-picker
-
Upload Images to Firebase Storage:
import { launchImageLibrary } from 'react-native-image-picker';
import 'firebase/storage';
const uploadImage = async () => {
const result = await launchImageLibrary({ mediaType: 'photo' });
if (result.assets) {
const { uri } = result.assets[0];
const response = await fetch(uri);
const blob = await response.blob();
const storageRef = firebase.storage().ref(`images/${Date.now()}`);
await storageRef.put(blob);
const downloadURL = await storageRef.getDownloadURL();
sendMessage(downloadURL, 'image');
}
};
const sendMessage = (content, type = 'text') => {
const messageRef = firebase.database().ref('messages');
const newMessage = {
content,
type,
timestamp: firebase.database.ServerValue.TIMESTAMP,
};
messageRef.push(newMessage);
setMessage('');
};
Step 8: Test Your Application
Run your application using Expo to test it on both Android and iOS devices:
expo start
Use the Expo Go app on your mobile device to scan the QR code and test the functionality.
Step 9: Deploy and Scale
Once development and testing are completed, consider deploying your backend to a cloud service like Google Cloud or AWS for scalability. Optimize your application for performance and security.
Get Started with Your Chat App!
Explore our step-by-step guide to create a chat application like WhatsApp. Dive into real-time messaging, user authentication, and more!
Conclusion
Are you considering to develop your web app, that highly resembles whatsapp features? Remember that developing a chat application like WhatsApp is both a challenging and rewarding project. By following this guide, you've laid the groundwork for a real-time messaging platform with essential features like user authentication, instant messaging, and media sharing.
As you continue to develop and refine your app, remember that the possibilities are endless, consider exploring advanced features like voice and video calls or adding robust encryption for enhanced security.
With your new skills and a solid foundation, you're well on your way to building a compelling chat experience that users will love. Happy coding!
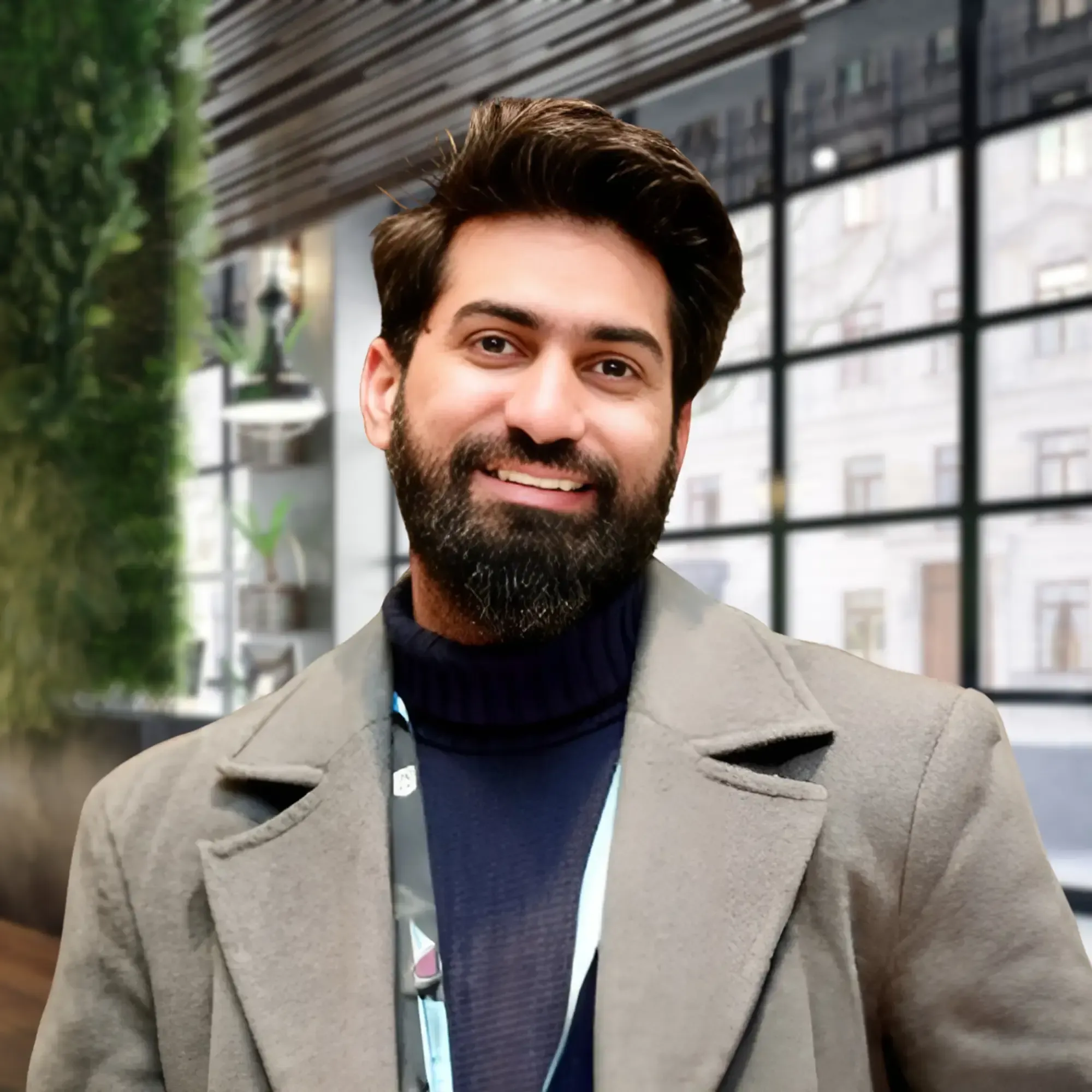
Written by :
Furqan Aziz
Furqan Aziz is CEO & Founder of InvoTeams. He is a tech enthusiast by heart with 10+ years of development experience & occasionally writes about technology here.