Table of Contents
In web development, building a robust and user-friendly admin panel can be time-consuming and complex at the same time. Even if you're managing user data, overseeing application content, or configuring system settings, an effective admin panel is crucial for streamlined operations.
Fortunately, AdminJS, an open-source library, simplifies this task significantly. Designed specifically for Node.js applications, AdminJS enables developers to set up a powerful and customizable admin dashboard quickly and efficiently.
AdminJS stands out with its intuitive interface and extensive customization options, making it ideal for developers who need to get an admin panel up and running without reinventing the wheel. With easy integration into popular databases and frameworks, AdminJS allows you to focus on your core application logic while it takes care of the administrative interface.
In this guide, we'll shed light on the process of integrating AdminJS into a Node.js project using Express and MongoDB. You'll learn how to set up basic CRUD operations, customize the admin panel to suit your needs, and ensure that your admin dashboard is both functional and secure.
Prerequisites
Before starting, ensure you have the following:
- Node.js Installed: Download and install Node.js from Node.js.
- Express Framework: We'll use Express to create the server, though AdminJS supports other frameworks as well.
- Basic Knowledge of JavaScript and Node.js: Familiarity with Node.js and JavaScript will be helpful.
Step 1: Setting Up the Node.js Project
Start by creating a new Node.js project and installing the necessary dependencies:
mkdir admin-panel
cd admin-panel
npm init -y
This creates a new directory and initializes it with a package.json file.
Step 2: Install Required Packages
Next, you’ll need to install AdminJS and the necessary adapter for your database (we’ll use MongoDB and Mongoose in this example). Also, install express and express-formidable for handling the server and file uploads:
npm install express mongoose adminjs @adminjs/express @adminjs/mongoose express-formidable
Step 3: Set Up Your Express Server
Create a new file index.js and set up a basic Express server:
const express = require('express');
const mongoose = require('mongoose');
const AdminJS = require('adminjs');
const AdminJSExpress = require('@adminjs/express');
const AdminJSMongoose = require('@adminjs/mongoose');
const app = express();
const PORT = 3000;
// Connect to MongoDB
mongoose.connect('mongodb://localhost:27017/adminjs-example', {
useNewUrlParser: true,
useUnifiedTopology: true,
});
// Define a simple Mongoose model
const User = mongoose.model('User', {
name: String,
email: String,
password: String,
});
// Initialize AdminJS
AdminJS.registerAdapter(AdminJSMongoose);
const adminJs = new AdminJS({
resources: [User],
rootPath: '/admin',
});
// Build and use the router
const router = AdminJSExpress.buildRouter(adminJs);
app.use(adminJs.options.rootPath, router);
// Start the Express server
app.listen(PORT, () => {
console.log(`Server is running on http://localhost:${PORT}`);
});
This code does the following:
- Connect to MongoDB: Establishes a connection to a local MongoDB instance.
- Defines a Mongoose Model: Creates a simple User model with fields for name, email, and password.
- Initializes AdminJS: AdminJS is registered with the Mongoose adapter, and the User model is passed as a resource.
- Sets Up the Admin Panel: The admin panel is accessible at /admin.
- Starts the Server: The server listens on port 3000.
Step 4: Running the Application
To start your admin panel, run:
node index.js
Open your browser and navigate to http://localhost:3000/admin. You should see the AdminJS interface with the User model available for CRUD operations.
Step 5: Customizing the Admin Panel
AdminJS allows you to customize the interface and behavior to suit your needs. Here's how you can customize fields, add actions, and set up authentication.
-
Customize Fields:
You might not want all fields to be visible or editable in the admin panel. You can customize the resource by specifying properties:
const adminJs = new AdminJS({
resources: [
{
resource: User,
options: {
properties: {
password: { isVisible: { list: false, edit: true, filter: false, show: false } },
},
},
},
],
rootPath: '/admin',
});
This code hides the password field in the list and show views but keeps it editable.
-
Add Actions:
AdminJS allows you to add custom actions to your resources. For example, you can add an action to send a welcome email to a new user:
const adminJs = new AdminJS({
resources: [
{
resource: User,
options: {
actions: {
sendWelcomeEmail: {
actionType: 'record',
icon: 'Send',
handler: async (request, response, context) => {
const user = context.record.toJSON();
// Code to send email
console.log(`Sending welcome email to ${user.email}`);
return {
record: context.record.toJSON(),
};
},
},
},
},
},
],
rootPath: '/admin',
});
This action, when triggered, logs a message to the console indicating an email is being sent.
-
Set Up Authentication:
To secure your admin panel, you can set up basic authentication:
const router = AdminJSExpress.buildAuthenticatedRouter(adminJs, {
authenticate: async (email, password) => {
// Replace with your authentication logic
if (email === 'admin@example.com' && password === 'password') {
return { email };
}
return null;
},
cookiePassword: 'some-secret-password-used-to-secure-cookie',
});
app.use(adminJs.options.rootPath, router);
This example uses a simple hardcoded authentication check, but you should replace it with real authentication logic, such as checking against a database.
Step 6: Areas to Be Careful About
- Security: Always implement proper authentication and authorization checks before deploying your admin panel to production.
- Sensitive Data: Be careful about exposing sensitive data in the admin panel. Use AdminJS's configuration options to hide or protect such fields.
- Database Performance: AdminJS generates queries dynamically, so ensure your database is optimized, especially when dealing with large datasets.
Conclusion
By now, you've learned how to harness the power of AdminJS to set up a fully functional admin panel for your Node.js application. From establishing a connection to MongoDB and configuring Mongoose models to customizing fields, adding actions, and setting up authentication, you have the foundational knowledge needed to create a versatile and secure admin dashboard.
AdminJS’s flexibility allows you to extend the admin panel’s functionality to meet your specific requirements, whether it’s through custom actions, field configurations, or additional resources. Be sure to explore the AdminJS documentation for more advanced customization options.
As you move forward, remember to keep security and performance in mind. Implement robust authentication mechanisms and optimize your database queries to ensure that your admin panel remains reliable and secure. AdminJS provides a solid foundation, but it's up to you to build upon it to create a tool that meets your unique requirements.
Explore the AdminJS documentation for more advanced features and customization options to further enhance your admin panel. With AdminJS, you build an admin interface and also create a vital component that will help you manage and scale your application effectively. Happy coding!
Table of Contents
In web development, building a robust and user-friendly admin panel can be time-consuming and complex at the same time. Even if you're managing user data, overseeing application content, or configuring system settings, an effective admin panel is crucial for streamlined operations.
Fortunately, AdminJS, an open-source library, simplifies this task significantly. Designed specifically for Node.js applications, AdminJS enables developers to set up a powerful and customizable admin dashboard quickly and efficiently.
AdminJS stands out with its intuitive interface and extensive customization options, making it ideal for developers who need to get an admin panel up and running without reinventing the wheel. With easy integration into popular databases and frameworks, AdminJS allows you to focus on your core application logic while it takes care of the administrative interface.
In this guide, we'll shed light on the process of integrating AdminJS into a Node.js project using Express and MongoDB. You'll learn how to set up basic CRUD operations, customize the admin panel to suit your needs, and ensure that your admin dashboard is both functional and secure.
Prerequisites
Before starting, ensure you have the following:
- Node.js Installed: Download and install Node.js from Node.js.
- Express Framework: We'll use Express to create the server, though AdminJS supports other frameworks as well.
- Basic Knowledge of JavaScript and Node.js: Familiarity with Node.js and JavaScript will be helpful.
Step 1: Setting Up the Node.js Project
Start by creating a new Node.js project and installing the necessary dependencies:
mkdir admin-panel
cd admin-panel
npm init -y
This creates a new directory and initializes it with a package.json file.
Step 2: Install Required Packages
Next, you’ll need to install AdminJS and the necessary adapter for your database (we’ll use MongoDB and Mongoose in this example). Also, install express and express-formidable for handling the server and file uploads:
npm install express mongoose adminjs @adminjs/express @adminjs/mongoose express-formidable
Step 3: Set Up Your Express Server
Create a new file index.js and set up a basic Express server:
const express = require('express');
const mongoose = require('mongoose');
const AdminJS = require('adminjs');
const AdminJSExpress = require('@adminjs/express');
const AdminJSMongoose = require('@adminjs/mongoose');
const app = express();
const PORT = 3000;
// Connect to MongoDB
mongoose.connect('mongodb://localhost:27017/adminjs-example', {
useNewUrlParser: true,
useUnifiedTopology: true,
});
// Define a simple Mongoose model
const User = mongoose.model('User', {
name: String,
email: String,
password: String,
});
// Initialize AdminJS
AdminJS.registerAdapter(AdminJSMongoose);
const adminJs = new AdminJS({
resources: [User],
rootPath: '/admin',
});
// Build and use the router
const router = AdminJSExpress.buildRouter(adminJs);
app.use(adminJs.options.rootPath, router);
// Start the Express server
app.listen(PORT, () => {
console.log(`Server is running on http://localhost:${PORT}`);
});
This code does the following:
- Connect to MongoDB: Establishes a connection to a local MongoDB instance.
- Defines a Mongoose Model: Creates a simple User model with fields for name, email, and password.
- Initializes AdminJS: AdminJS is registered with the Mongoose adapter, and the User model is passed as a resource.
- Sets Up the Admin Panel: The admin panel is accessible at /admin.
- Starts the Server: The server listens on port 3000.
Step 4: Running the Application
To start your admin panel, run:
node index.js
Open your browser and navigate to http://localhost:3000/admin. You should see the AdminJS interface with the User model available for CRUD operations.
Step 5: Customizing the Admin Panel
AdminJS allows you to customize the interface and behavior to suit your needs. Here's how you can customize fields, add actions, and set up authentication.
-
Customize Fields:
You might not want all fields to be visible or editable in the admin panel. You can customize the resource by specifying properties:
const adminJs = new AdminJS({
resources: [
{
resource: User,
options: {
properties: {
password: { isVisible: { list: false, edit: true, filter: false, show: false } },
},
},
},
],
rootPath: '/admin',
});
This code hides the password field in the list and show views but keeps it editable.
-
Add Actions:
AdminJS allows you to add custom actions to your resources. For example, you can add an action to send a welcome email to a new user:
const adminJs = new AdminJS({
resources: [
{
resource: User,
options: {
actions: {
sendWelcomeEmail: {
actionType: 'record',
icon: 'Send',
handler: async (request, response, context) => {
const user = context.record.toJSON();
// Code to send email
console.log(`Sending welcome email to ${user.email}`);
return {
record: context.record.toJSON(),
};
},
},
},
},
},
],
rootPath: '/admin',
});
This action, when triggered, logs a message to the console indicating an email is being sent.
-
Set Up Authentication:
To secure your admin panel, you can set up basic authentication:
const router = AdminJSExpress.buildAuthenticatedRouter(adminJs, {
authenticate: async (email, password) => {
// Replace with your authentication logic
if (email === 'admin@example.com' && password === 'password') {
return { email };
}
return null;
},
cookiePassword: 'some-secret-password-used-to-secure-cookie',
});
app.use(adminJs.options.rootPath, router);
This example uses a simple hardcoded authentication check, but you should replace it with real authentication logic, such as checking against a database.
Step 6: Areas to Be Careful About
- Security: Always implement proper authentication and authorization checks before deploying your admin panel to production.
- Sensitive Data: Be careful about exposing sensitive data in the admin panel. Use AdminJS's configuration options to hide or protect such fields.
- Database Performance: AdminJS generates queries dynamically, so ensure your database is optimized, especially when dealing with large datasets.
Conclusion
By now, you've learned how to harness the power of AdminJS to set up a fully functional admin panel for your Node.js application. From establishing a connection to MongoDB and configuring Mongoose models to customizing fields, adding actions, and setting up authentication, you have the foundational knowledge needed to create a versatile and secure admin dashboard.
AdminJS’s flexibility allows you to extend the admin panel’s functionality to meet your specific requirements, whether it’s through custom actions, field configurations, or additional resources. Be sure to explore the AdminJS documentation for more advanced customization options.
As you move forward, remember to keep security and performance in mind. Implement robust authentication mechanisms and optimize your database queries to ensure that your admin panel remains reliable and secure. AdminJS provides a solid foundation, but it's up to you to build upon it to create a tool that meets your unique requirements.
Explore the AdminJS documentation for more advanced features and customization options to further enhance your admin panel. With AdminJS, you build an admin interface and also create a vital component that will help you manage and scale your application effectively. Happy coding!
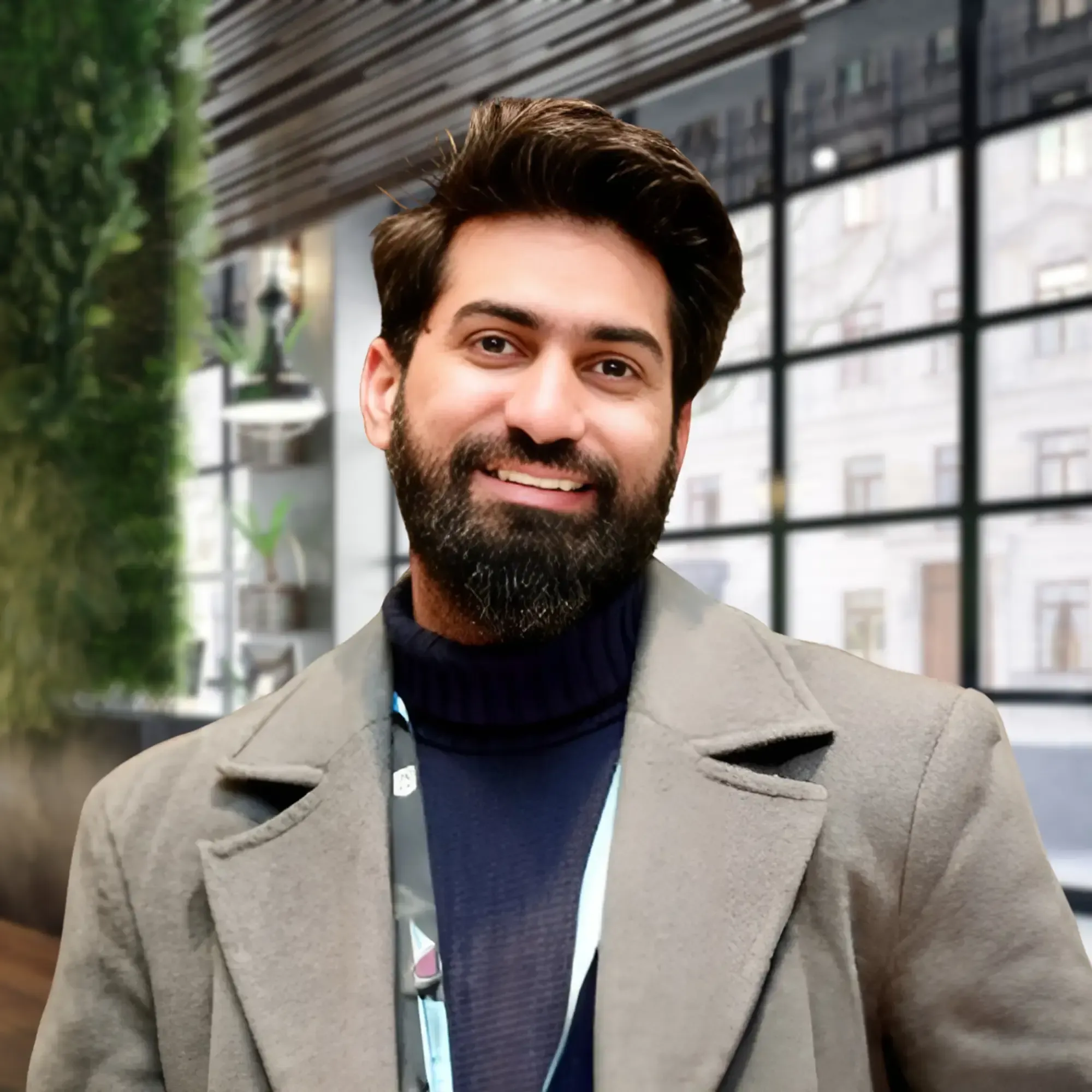
Written by :
Furqan Aziz
Furqan Aziz is CEO & Founder of InvoTeams. He is a tech enthusiast by heart with 10+ years of development experience & occasionally writes about technology here.