Table of Contents
- Want To See Your ChatBot In Action?
- What You’ll Get From This Guide?
- Why Build an AI-Based Chatbot?
- Prerequisites
- Step 1: Set Up Your Development Environment
- Step 2: Collect and Preprocess Data
- Step 3: Build the Chatbot Model
- Step 4: Implement Chatbot Responses
- Step 5: Enhance with NLP Techniques
- Conclusion
AI chatbots are the future of customer interaction. Discover how to build your own using Python and machine learning. Ready to create a chatbot that can hold its own? Join us as we explore the process of building one with Python.
Consider having a virtual assistant that can handle queries around the clock, or a support tool that simplifies complex interactions. In this guide, we’ll show you the process of building your very own chatbot using Python.
No matter if you’re a tech enthusiast or a business owner, this step-by-step guide will help you create a chatbot that’s both intelligent and engaging. Ready to dive in? Let’s get started!
AI-based chatbots are revolutionizing how businesses interact with their customers by providing instant support and personalized experiences. In this guide, we'll walk you through the process of creating a chatbot using Python and machine learning techniques, you can get AI services that are best among the rest to build these sort of chatbots.
Want To See Your ChatBot In Action?
Book a Demo Now
What You’ll Get From This Guide?
- Learning to Setup Your Environment: Install Python, set up a virtual environment, and add essential libraries.
- Data Collection & Preprocessing: Gather conversational data, and use NLTK to clean and tokenize text.
- Build the Model: Create a neural network with TensorFlow to process and understand user input.
- Generate Responses: Implement a function to produce replies based on user queries.
- Advanced Features: Enhance your chatbot with NLP techniques and pre-trained models for more human-like interactions.
Why Build an AI-Based Chatbot?
AI chatbots can enhance customer engagement, streamline operations, and provide 24/7 support. Whether you're looking to automate customer service or create a personal assistant, building a chatbot is a valuable addition to your tech stack.
Prerequisites
Before you start, ensure you have the following:
- Basic understanding of Python programming.
- Python 3.x installed on your machine.
- Access to the internet for downloading libraries and datasets.
Step 1: Set Up Your Development Environment
- Install Python 3.x from the official Python website.
- Set up a virtual environment for your project:
python3 -m venv chatbot-env
source chatbot-env/bin/activate # On Windows use `chatbot-env\Scripts\activate`
-
Install necessary libraries:
pip install nltk numpy scikit-learn tensorflow
Step 2: Collect and Preprocess Data
- Collect a dataset of conversational data. You can use publicly available datasets like the Cornell Movie-Dialogs Corpus.
- Preprocess the data using NLTK:
import nltk
nltk.download('punkt')
from nltk.tokenize import word_tokenize
def preprocess_text(text):
return word_tokenize(text.lower())
-
Using Pre-trained Word Embeddings:
from gensim.models import Word2Vec
import numpy as np
corpus = ['Hello, how can I help you?', 'What is your name?', 'How do you work?']
tokenized_corpus = [preprocess_text(sentence) for sentence in corpus]
embedding_model = Word2Vec(tokenized_corpus, vector_size=100, window=5, min_count=1, workers=4) word_vectors = embedding_model.wv
def get_embedding(text):
tokens = preprocess_text(text)
return np.mean([word_vectors[word] for word in tokens if word in word_vectors], axis=0)
X = np.array([get_embedding(sentence) for sentence in corpus])
By using word embeddings, the chatbot gains a deeper understanding of language, enabling it to respond more accurately to diverse user inputs.
Step 3: Build the Chatbot Model
-
Choose a machine learning model. Here, we’ll use a simple neural network with TensorFlow:
import tensorflow as tf
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Dense
model = Sequential([
Dense(128, input_shape=(X.shape[1],), activation='relu'),
Dense(64, activation='relu'),
Dense(len(corpus), activation='softmax')
])
model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
-
Train your model:
from sklearn.preprocessing import OneHotEncoder
encoder = OneHotEncoder()
y = encoder.fit_transform([[i] for i in range(len(corpus))]).toarray()
model.fit(X.toarray(), y, epochs=100, batch_size=8)
Step 4: Implement Chatbot Responses
-
Create a function to generate responses based on user input:
def get_response(user_input):
user_vector = get_embedding(user_input)
prediction = model.predict(np.array([user_vector]))
response_index = prediction.argmax()
return corpus[response_index]
while True:
user_input = input("You: ")
if user_input.lower() == 'exit':
break
print("Bot:", get_response(user_input))
Step 5: Enhance with NLP Techniques
-
For a more robust chatbot, consider integrating advanced NLP techniques like sentiment analysis, named entity recognition, or using pre-trained models such as GPT-3 or BERT. These models can significantly enhance the chatbot's ability to understand and generate human-like responses.
For example, you can replace the simple neural network with a transformer-based model:
from transformers import pipeline
chatbot = pipeline('conversational', model='microsoft/DialoGPT-medium')
response = chatbot("How can I help you?")
print(response)
Conclusion
From setting up your development environment to training your model, you’ve covered the essential steps to create a functional chatbot. As you continue to refine your bot, consider exploring advanced techniques and tools like sentiment analysis and transformer-based models to elevate its capabilities. The possibilities are endless, and your chatbot can grow to handle increasingly sophisticated interactions. Keep experimenting and learning, your journey into the world of AI has just begun!
If you're interested in exploring advanced AI and machine learning solutions for your business, discover our AI/ML services to see how we can help you harness the power of AI.
Table of Contents
- Want To See Your ChatBot In Action?
- What You’ll Get From This Guide?
- Why Build an AI-Based Chatbot?
- Prerequisites
- Step 1: Set Up Your Development Environment
- Step 2: Collect and Preprocess Data
- Step 3: Build the Chatbot Model
- Step 4: Implement Chatbot Responses
- Step 5: Enhance with NLP Techniques
- Conclusion
AI chatbots are the future of customer interaction. Discover how to build your own using Python and machine learning. Ready to create a chatbot that can hold its own? Join us as we explore the process of building one with Python.
Consider having a virtual assistant that can handle queries around the clock, or a support tool that simplifies complex interactions. In this guide, we’ll show you the process of building your very own chatbot using Python.
No matter if you’re a tech enthusiast or a business owner, this step-by-step guide will help you create a chatbot that’s both intelligent and engaging. Ready to dive in? Let’s get started!
AI-based chatbots are revolutionizing how businesses interact with their customers by providing instant support and personalized experiences. In this guide, we'll walk you through the process of creating a chatbot using Python and machine learning techniques, you can get AI services that are best among the rest to build these sort of chatbots.
Want To See Your ChatBot In Action?
Book a Demo Now
What You’ll Get From This Guide?
- Learning to Setup Your Environment: Install Python, set up a virtual environment, and add essential libraries.
- Data Collection & Preprocessing: Gather conversational data, and use NLTK to clean and tokenize text.
- Build the Model: Create a neural network with TensorFlow to process and understand user input.
- Generate Responses: Implement a function to produce replies based on user queries.
- Advanced Features: Enhance your chatbot with NLP techniques and pre-trained models for more human-like interactions.
Why Build an AI-Based Chatbot?
AI chatbots can enhance customer engagement, streamline operations, and provide 24/7 support. Whether you're looking to automate customer service or create a personal assistant, building a chatbot is a valuable addition to your tech stack.
Prerequisites
Before you start, ensure you have the following:
- Basic understanding of Python programming.
- Python 3.x installed on your machine.
- Access to the internet for downloading libraries and datasets.
Step 1: Set Up Your Development Environment
- Install Python 3.x from the official Python website.
- Set up a virtual environment for your project:
python3 -m venv chatbot-env
source chatbot-env/bin/activate # On Windows use `chatbot-env\Scripts\activate`
-
Install necessary libraries:
pip install nltk numpy scikit-learn tensorflow
Step 2: Collect and Preprocess Data
- Collect a dataset of conversational data. You can use publicly available datasets like the Cornell Movie-Dialogs Corpus.
- Preprocess the data using NLTK:
import nltk
nltk.download('punkt')
from nltk.tokenize import word_tokenize
def preprocess_text(text):
return word_tokenize(text.lower())
-
Using Pre-trained Word Embeddings:
from gensim.models import Word2Vec
import numpy as np
corpus = ['Hello, how can I help you?', 'What is your name?', 'How do you work?']
tokenized_corpus = [preprocess_text(sentence) for sentence in corpus]
embedding_model = Word2Vec(tokenized_corpus, vector_size=100, window=5, min_count=1, workers=4) word_vectors = embedding_model.wv
def get_embedding(text):
tokens = preprocess_text(text)
return np.mean([word_vectors[word] for word in tokens if word in word_vectors], axis=0)
X = np.array([get_embedding(sentence) for sentence in corpus])
By using word embeddings, the chatbot gains a deeper understanding of language, enabling it to respond more accurately to diverse user inputs.
Step 3: Build the Chatbot Model
-
Choose a machine learning model. Here, we’ll use a simple neural network with TensorFlow:
import tensorflow as tf
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Dense
model = Sequential([
Dense(128, input_shape=(X.shape[1],), activation='relu'),
Dense(64, activation='relu'),
Dense(len(corpus), activation='softmax')
])
model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy'])
-
Train your model:
from sklearn.preprocessing import OneHotEncoder
encoder = OneHotEncoder()
y = encoder.fit_transform([[i] for i in range(len(corpus))]).toarray()
model.fit(X.toarray(), y, epochs=100, batch_size=8)
Step 4: Implement Chatbot Responses
-
Create a function to generate responses based on user input:
def get_response(user_input):
user_vector = get_embedding(user_input)
prediction = model.predict(np.array([user_vector]))
response_index = prediction.argmax()
return corpus[response_index]
while True:
user_input = input("You: ")
if user_input.lower() == 'exit':
break
print("Bot:", get_response(user_input))
Step 5: Enhance with NLP Techniques
-
For a more robust chatbot, consider integrating advanced NLP techniques like sentiment analysis, named entity recognition, or using pre-trained models such as GPT-3 or BERT. These models can significantly enhance the chatbot's ability to understand and generate human-like responses.
For example, you can replace the simple neural network with a transformer-based model:
from transformers import pipeline
chatbot = pipeline('conversational', model='microsoft/DialoGPT-medium')
response = chatbot("How can I help you?")
print(response)
Conclusion
From setting up your development environment to training your model, you’ve covered the essential steps to create a functional chatbot. As you continue to refine your bot, consider exploring advanced techniques and tools like sentiment analysis and transformer-based models to elevate its capabilities. The possibilities are endless, and your chatbot can grow to handle increasingly sophisticated interactions. Keep experimenting and learning, your journey into the world of AI has just begun!
If you're interested in exploring advanced AI and machine learning solutions for your business, discover our AI/ML services to see how we can help you harness the power of AI.
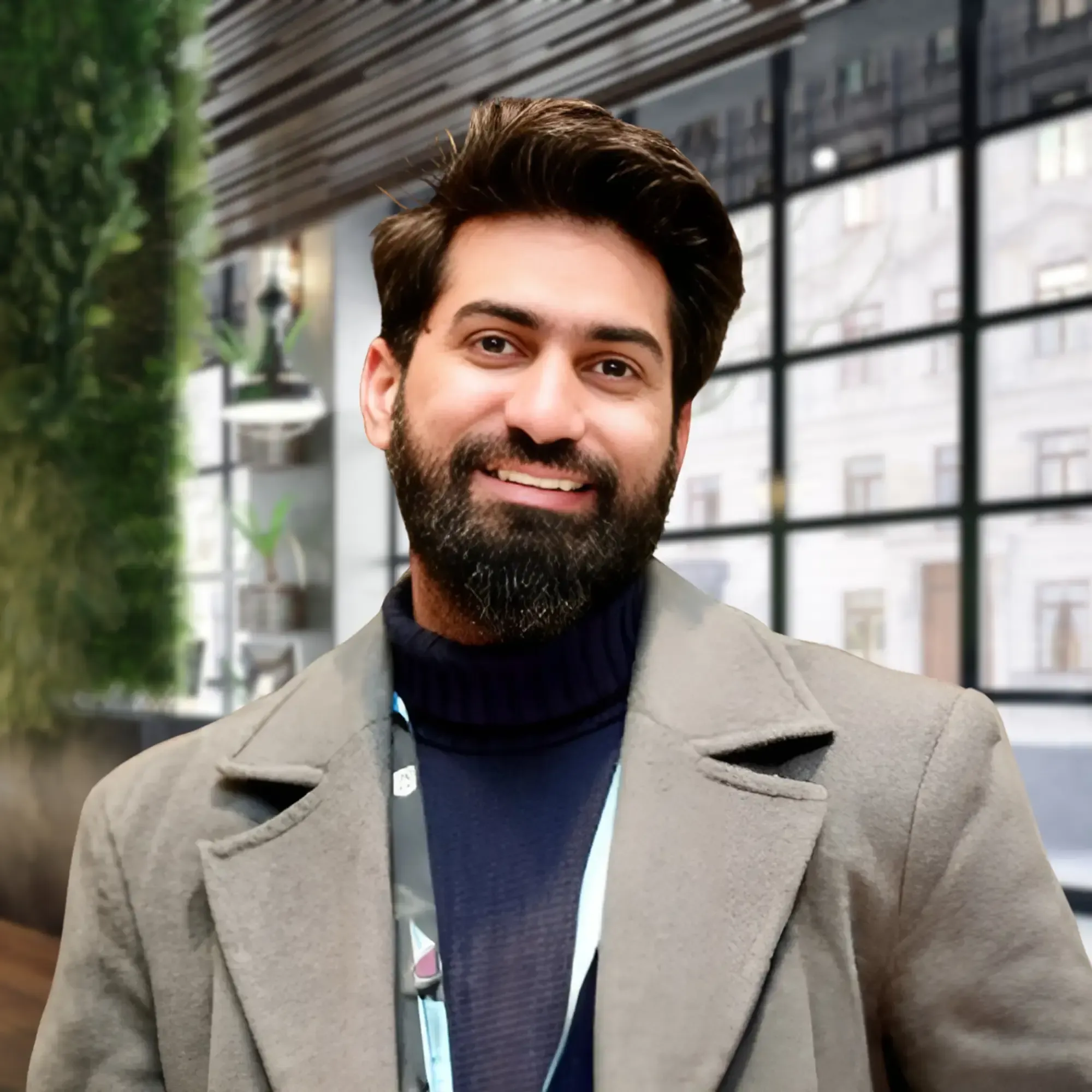
Written by :
Furqan Aziz
Furqan Aziz is CEO & Founder of InvoTeams. He is a tech enthusiast by heart with 10+ years of development experience & occasionally writes about technology here.