Table of Contents
Learn how to build a fun and interactive “Tap Tap” game on Telegram with this straightforward guide.
Here in this must-read blog, you'll discover how to create an engaging “Tap Tap” mini-game that keeps users tapping and having a blast.
With Telegram’s powerful bot framework at your fingertips, you’ll explore how to set up your bot, code the game mechanics, and deliver an entertaining experience right within the messaging app. Everything you ever wanted to learn is right here.
If you’re excited about building interactive experiences or just curious about bot development, this guide will provide you with the steps to get started.
Prerequisites
Before you begin, ensure you have the following:
- Telegram Account: You need a Telegram account to create and manage your bot.
- Node.js Installed: Ensure Node.js is installed on your machine. You can download it from Node.js.
- Basic Knowledge of JavaScript and Node.js: Familiarity with JavaScript and Node.js will be beneficial.
- BotFather: Access to the Telegram BotFather, which you’ll use to create and manage your bot.
Step 1: Creating a Telegram Bot
First, create a new bot using Telegram’s BotFather:
- Open Telegram and search for "BotFather".
- Start a conversation and use the command /newbot.
- Follow the prompts to give your bot a name and username.
- BotFather will provide you with a token, which you'll need to interact with the Telegram API.
Step 2: Setting Up Your Node.js Project
Create a new directory for your project and initialize it with npm:
mkdir tap-tap-telegram
cd tap-tap-telegram
npm init -y
This creates a new directory and initializes it with a package.json file.
Step 3: Install Required Packages
Install the node-telegram-bot-api package, which simplifies interactions with the Telegram API:
npm install node-telegram-bot-api
Step 4: Set Up the Telegram Bot
In the project directory, create a new file index.js. This file will handle the bot's setup and interactions.
-
Import Required Modules:
const TelegramBot = require('node-telegram-bot-api');
The node-telegram-bot-api module is imported to interact with Telegram’s API.
-
Initialize the Bot:
const token = 'YOUR_TELEGRAM_BOT_TOKEN';
const bot = new TelegramBot(token, { polling: true });
Replace YOUR_TELEGRAM_BOT_TOKEN with the token you received from BotFather.
-
Set Up Basic Commands:
Add a simple start command that users can use to initiate the game:
bot.onText(/\/start/, (msg) => {
bot.sendMessage(msg.chat.id, "Welcome to Tap Tap Game! Type /play to start tapping.");
});
This command will welcome the user and prompt them to start the game.
Step 5: Developing the Tap Tap Game Logic
Now, let's add the game logic. The idea is simple: users will tap (send messages), and the bot will count and respond based on their taps.
-
Game State Management:
You’ll need to track the user's progress. For simplicity, use an in-memory object:
let gameState = {};
- Start the Game:
Add a command to start the game and initialize the user's state:
bot.onText(/\/play/, (msg) => {
const chatId = msg.chat.id;
gameState[chatId] = { taps: 0 };
bot.sendMessage(chatId, "Game started! Tap as fast as you can. Type /tap to tap.");
});
This command initializes the user's game state and prompts them to start tapping.
-
Handle Taps:
Create a command that increments the tap count each time the user sends a /tap command:
bot.onText(/\/tap/, (msg) => {
const chatId = msg.chat.id;
if (!gameState[chatId]) {
bot.sendMessage(chatId, "Type /play to start the game first.");
return;
}
gameState[chatId].taps += 1;
bot.sendMessage(chatId, `You've tapped ${gameState[chatId].taps} times! Keep going!`);
});
This command updates the user's tap count and provides feedback.
-
End the Game:
You might want to add a condition to end the game after a certain number of taps or a timer:
bot.onText(/\/end/, (msg) => {
const chatId = msg.chat.id;
if (!gameState[chatId]) {
bot.sendMessage(chatId, "There's no game in progress. Type /play to start.");
return;
}
const taps = gameState[chatId].taps;
delete gameState[chatId];
bot.sendMessage(chatId, `Game over! You tapped ${taps} times.`);
});
This command ends the game and displays the user's final tap count.
Step 6: Running the Bot
To start your bot, run:
node index.js
Open Telegram and interact with your bot by typing /start, /play, /tap, and /end to see how the game works.
Step 7: Areas to Be Careful About
- Bot Polling: The bot is set up with polling, which is fine for development. For production, consider setting up a webhook to handle messages more efficiently.
- Game State Persistence: The game state is stored in memory, which means it will be lost if the bot restarts. Consider using a database for persistence if you plan to scale.
- Rate Limiting: Be aware of Telegram's message rate limits to avoid your bot being temporarily blocked for sending too many messages.
Conclusion
You've now built a lively “Tap Tap” mini-game on Telegram! By following these instructions, you’ve set up a functional bot and crafted a game that users can enjoy.
But the journey doesn't end here. There's a wide range of possibilities to explore, from adding features like leaderboards and time-based challenges to incorporating multimedia elements and multiplayer modes.
The basics are a great start, but there's always room to expand and innovate. As you continue to develop and refine your game, consider how you can further engage your users and enhance their experience. Keep experimenting and pushing the boundaries of what your Telegram bot can do. Your creativity and ingenuity can turn a simple game into a standout feature that users love to interact with.
Table of Contents
Learn how to build a fun and interactive “Tap Tap” game on Telegram with this straightforward guide.
Here in this must-read blog, you'll discover how to create an engaging “Tap Tap” mini-game that keeps users tapping and having a blast.
With Telegram’s powerful bot framework at your fingertips, you’ll explore how to set up your bot, code the game mechanics, and deliver an entertaining experience right within the messaging app. Everything you ever wanted to learn is right here.
If you’re excited about building interactive experiences or just curious about bot development, this guide will provide you with the steps to get started.
Prerequisites
Before you begin, ensure you have the following:
- Telegram Account: You need a Telegram account to create and manage your bot.
- Node.js Installed: Ensure Node.js is installed on your machine. You can download it from Node.js.
- Basic Knowledge of JavaScript and Node.js: Familiarity with JavaScript and Node.js will be beneficial.
- BotFather: Access to the Telegram BotFather, which you’ll use to create and manage your bot.
Step 1: Creating a Telegram Bot
First, create a new bot using Telegram’s BotFather:
- Open Telegram and search for "BotFather".
- Start a conversation and use the command /newbot.
- Follow the prompts to give your bot a name and username.
- BotFather will provide you with a token, which you'll need to interact with the Telegram API.
Step 2: Setting Up Your Node.js Project
Create a new directory for your project and initialize it with npm:
mkdir tap-tap-telegram
cd tap-tap-telegram
npm init -y
This creates a new directory and initializes it with a package.json file.
Step 3: Install Required Packages
Install the node-telegram-bot-api package, which simplifies interactions with the Telegram API:
npm install node-telegram-bot-api
Step 4: Set Up the Telegram Bot
In the project directory, create a new file index.js. This file will handle the bot's setup and interactions.
-
Import Required Modules:
const TelegramBot = require('node-telegram-bot-api');
The node-telegram-bot-api module is imported to interact with Telegram’s API.
-
Initialize the Bot:
const token = 'YOUR_TELEGRAM_BOT_TOKEN';
const bot = new TelegramBot(token, { polling: true });
Replace YOUR_TELEGRAM_BOT_TOKEN with the token you received from BotFather.
-
Set Up Basic Commands:
Add a simple start command that users can use to initiate the game:
bot.onText(/\/start/, (msg) => {
bot.sendMessage(msg.chat.id, "Welcome to Tap Tap Game! Type /play to start tapping.");
});
This command will welcome the user and prompt them to start the game.
Step 5: Developing the Tap Tap Game Logic
Now, let's add the game logic. The idea is simple: users will tap (send messages), and the bot will count and respond based on their taps.
-
Game State Management:
You’ll need to track the user's progress. For simplicity, use an in-memory object:
let gameState = {};
- Start the Game:
Add a command to start the game and initialize the user's state:
bot.onText(/\/play/, (msg) => {
const chatId = msg.chat.id;
gameState[chatId] = { taps: 0 };
bot.sendMessage(chatId, "Game started! Tap as fast as you can. Type /tap to tap.");
});
This command initializes the user's game state and prompts them to start tapping.
-
Handle Taps:
Create a command that increments the tap count each time the user sends a /tap command:
bot.onText(/\/tap/, (msg) => {
const chatId = msg.chat.id;
if (!gameState[chatId]) {
bot.sendMessage(chatId, "Type /play to start the game first.");
return;
}
gameState[chatId].taps += 1;
bot.sendMessage(chatId, `You've tapped ${gameState[chatId].taps} times! Keep going!`);
});
This command updates the user's tap count and provides feedback.
-
End the Game:
You might want to add a condition to end the game after a certain number of taps or a timer:
bot.onText(/\/end/, (msg) => {
const chatId = msg.chat.id;
if (!gameState[chatId]) {
bot.sendMessage(chatId, "There's no game in progress. Type /play to start.");
return;
}
const taps = gameState[chatId].taps;
delete gameState[chatId];
bot.sendMessage(chatId, `Game over! You tapped ${taps} times.`);
});
This command ends the game and displays the user's final tap count.
Step 6: Running the Bot
To start your bot, run:
node index.js
Open Telegram and interact with your bot by typing /start, /play, /tap, and /end to see how the game works.
Step 7: Areas to Be Careful About
- Bot Polling: The bot is set up with polling, which is fine for development. For production, consider setting up a webhook to handle messages more efficiently.
- Game State Persistence: The game state is stored in memory, which means it will be lost if the bot restarts. Consider using a database for persistence if you plan to scale.
- Rate Limiting: Be aware of Telegram's message rate limits to avoid your bot being temporarily blocked for sending too many messages.
Conclusion
You've now built a lively “Tap Tap” mini-game on Telegram! By following these instructions, you’ve set up a functional bot and crafted a game that users can enjoy.
But the journey doesn't end here. There's a wide range of possibilities to explore, from adding features like leaderboards and time-based challenges to incorporating multimedia elements and multiplayer modes.
The basics are a great start, but there's always room to expand and innovate. As you continue to develop and refine your game, consider how you can further engage your users and enhance their experience. Keep experimenting and pushing the boundaries of what your Telegram bot can do. Your creativity and ingenuity can turn a simple game into a standout feature that users love to interact with.
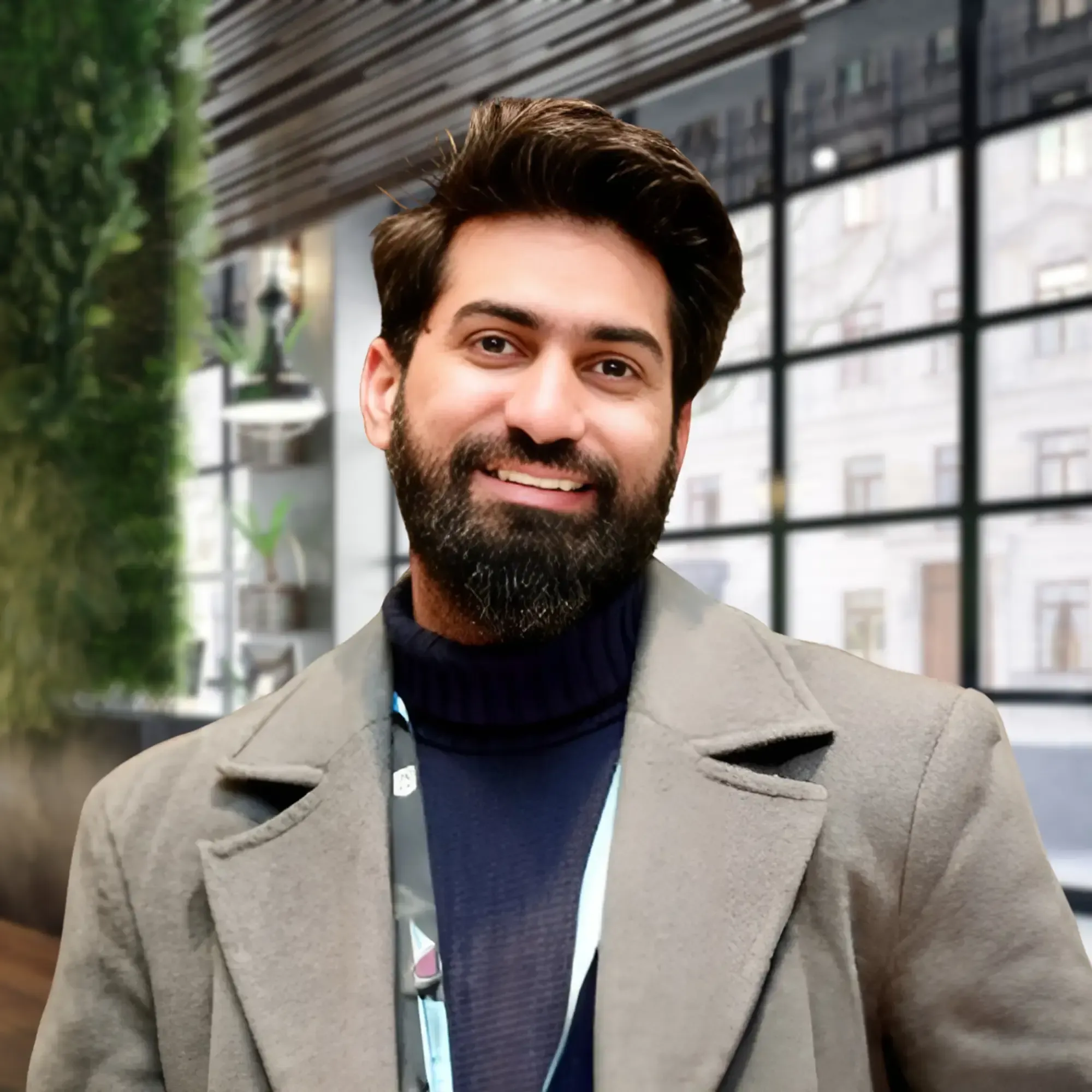
Written by :
Furqan Aziz
Furqan Aziz is CEO & Founder of InvoTeams. He is a tech enthusiast by heart with 10+ years of development experience & occasionally writes about technology here.