Table of Contents
In the rapidly advancing digital landscape, securing and verifying user identities efficiently is essential for meeting regulatory standards and building trust. Traditional KYC processes often involve lengthy procedures and physical documentation, which can slow down operations and increase the risk of errors.
eKYC, or electronic Know Your Customer, offers a modern solution by digitizing these verification steps, thus simplifying and securing the process. When integrated with blockchain technology, eKYC benefits from the added security, transparency, and immutability that blockchain provides.
This combination ensures that identity verification is not only more efficient but also more reliable. In this detailed guide, we will walk you through the entire process of incorporating an eKYC feature into a blockchain application.
From establishing your blockchain environment to coding and securing the eKYC functionality, this guide will provide you with the tools and knowledge needed to implement a robust and compliant verification system.
Integrate eKYC Processes With Blockchain Technology.
- Efficient User Verification: Streamline the process of verifying user identities with digital tools, reducing the time and effort required compared to traditional methods.
- Enhanced Security: Utilize blockchain’s robust security features to protect sensitive user data and ensure that verification processes are tamper-proof.
- Immutable Records: Store verification proofs on the blockchain for permanent, unchangeable records, providing a transparent and auditable trail of identity verification.
- Compliance Assurance: Meet regulatory requirements more effectively by leveraging digital records and automated processes that adhere to industry standards.
- Reduced Error Rates: Minimize the risk of human error and data discrepancies through automated verification and secure document storage.
Prerequisites
Before starting, ensure you have the following:
- Blockchain Environment: Set up a blockchain environment, such as Ethereum, Hyperledger, or a private blockchain.
- Node.js Installed: Ensure Node.js is installed on your machine.
- Smart Contract Development Tools: Tools like Truffle, Hardhat, or Remix for developing smart contracts.
- Basic Knowledge of Blockchain, Smart Contracts, and Node.js: Familiarity with these technologies will be helpful.
Step 1: Understanding eKYC Requirements
eKYC involves verifying the identity of users through digital means. The key components include:
- User Identity Verification: Collecting and verifying identity documents.
- Document Storage: Storing verified documents securely.
- Blockchain Integration: Storing verification proofs on the blockchain for immutability and transparency.
Step 2: Setting Up the Blockchain Environment
Start by setting up your blockchain environment. For this example, we'll use Ethereum and Truffle:
- Install Truffle and Ganache:
npm install -g truffle
npm install -g ganache-cli
-
Create a New Truffle Project:
truffle init eKYC-app
cd eKYC-app
This sets up a basic Truffle project structure.
-
Run Ganache:
Start a local Ethereum blockchain using Ganache:
ganache-cli
This local blockchain will simulate the Ethereum network for development purposes.
Step 3: Developing the Smart Contract
Create a smart contract to handle eKYC verification. In the contracts directory, create a new file KYC.sol:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
contract KYC {
struct User {
string name;
string documentHash;
bool isVerified;
}
mapping(address => User) public users;
address public admin;
modifier onlyAdmin() {
require(msg.sender == admin, "Only admin can perform this action");
_;
}
constructor() {
admin = msg.sender;
}
function addUser(string memory _name, string memory _documentHash) public {
users[msg.sender] = User(_name, _documentHash, false);
}
function verifyUser(address _userAddress) public onlyAdmin {
users[_userAddress].isVerified = true;
}
function isUserVerified(address _userAddress) public view returns (bool) {
return users[_userAddress].isVerified;
}
}
This smart contract does the following:
- User Structure: Defines a user structure with name, documentHash, and isVerified fields.
- Add User: Allows users to add their information and document hash.
- Verify User: Allows an admin to verify the user.
- Check Verification: Allows anyone to check if a user is verified.
Step 4: Deploying the Smart Contract
Next, deploy the smart contract to the local blockchain:
-
Create a Migration Script:
In the migrations directory, create a new file 2_deploy_kyc.js:
const KYC = artifacts.require("KYC");
module.exports = function (deployer) {
deployer.deploy(KYC);
};
-
Deploy the Contract:
Deploy the contract using Truffle:
truffle migrate --network development
This command deploys the KYC contract to the local blockchain.
Step 5: Integrating the eKYC Feature in a Node.js Backend
Now, let's set up a Node.js backend to interact with the smart contract and handle the eKYC process.
-
Set Up the Project:
In the project directory, initialize a Node.js project:
npm init -y
npm install web3 truffle-contract express body-parser
-
Create a Server File:
Create a new file server.js in the root directory:
const express = require('express');
const bodyParser = require('body-parser');
const Web3 = require('web3');
const contract = require('truffle-contract');
const KYCContract = require('./build/contracts/KYC.json');
const app = express();
app.use(bodyParser.json());
const web3 = new Web3(new Web3.providers.HttpProvider("http://localhost:8545"));
const KYC = contract(KYCContract);
KYC.setProvider(web3.currentProvider);
const adminAddress = "YOUR_ADMIN_ADDRESS"; // Replace with your admin address
app.post('/addUser', async (req, res) => {
const { name, documentHash, userAddress } = req.body;
try {
const instance = await KYC.deployed();
await instance.addUser(name, documentHash, { from: userAddress });
res.status(200).send("User added successfully");
} catch (error) {
res.status(500).send(error.toString());
}
});
app.post('/verifyUser', async (req, res) => {
const { userAddress } = req.body;
try {
const instance = await KYC.deployed();
await instance.verifyUser(userAddress, { from: adminAddress });
res.status(200).send("User verified successfully");
} catch (error) {
res.status(500).send(error.toString());
}
});
app.get('/isUserVerified/:userAddress', async (req, res) => {
const { userAddress } = req.params;
try {
const instance = await KYC.deployed();
const isVerified = await instance.isUserVerified(userAddress);
res.status(200).send({ isVerified });
} catch (error) {
res.status(500).send(error.toString());
}
});
app.listen(3000, () => {
console.log('Server running on port 3000');
});
This code does the following:
- Web3 Integration: Integrates Web3 to interact with the Ethereum blockchain.
- Express Server: Sets up an Express server to handle HTTP requests.
- User Management: Provides endpoints to add users, verify users, and check if a user is verified.
Step 6: Running and Testing the Application
To start your server and test the eKYC feature:
-
Start the Server:
node server.js
-
Test the Endpoints:
Use a tool like Postman to test the API endpoints:
-
Add User:
POST http://localhost:3000/addUser
Body: { "name": "John Doe", "documentHash": "Qm12345...", "userAddress": "0x123..." }
- Verify User:
POST http://localhost:3000/verifyUser
Body: { "userAddress": "0x123..." }
- Check Verification:
GET http://localhost:3000/isUserVerified/0x123...
Step 7: Areas to Be Careful About
- Data Privacy: Ensure that sensitive data such as user documents are stored securely and handled according to data protection regulations.
- Smart Contract Security: Write secure smart contracts to avoid vulnerabilities like reentrancy attacks or unauthorized access.
- Scalability: Consider the scalability of your eKYC solution, especially if you plan to deploy it on a public blockchain where transaction costs and speeds are variable.
Ready To Modernize Your Identity Verification Process?
Implement An eKyc Feature In Your Blockchain App And Ensure Secure, Efficient, And Compliant User Verification.
Conclusion
As digital platforms and regulations evolve, maintaining effective and compliant identity verification systems is crucial. By incorporating eKYC into your blockchain application, you’re adopting a forward-thinking approach that combines modern technology with rigorous security measures.
This guide has outlined the steps required to build an effective eKYC feature, including setting up your blockchain infrastructure, developing smart contracts, and integrating a backend system. The result is a sophisticated eKYC solution that ensures secure, transparent, and reliable user verification.
Moving forward, you may consider additional enhancements such as integrating with external KYC providers or implementing advanced security features to further fortify your system. By staying proactive and continually refining your approach, you’ll ensure your eKYC system remains robust and adaptable, meeting the evolving needs of both users and regulatory bodies.
Table of Contents
In the rapidly advancing digital landscape, securing and verifying user identities efficiently is essential for meeting regulatory standards and building trust. Traditional KYC processes often involve lengthy procedures and physical documentation, which can slow down operations and increase the risk of errors.
eKYC, or electronic Know Your Customer, offers a modern solution by digitizing these verification steps, thus simplifying and securing the process. When integrated with blockchain technology, eKYC benefits from the added security, transparency, and immutability that blockchain provides.
This combination ensures that identity verification is not only more efficient but also more reliable. In this detailed guide, we will walk you through the entire process of incorporating an eKYC feature into a blockchain application.
From establishing your blockchain environment to coding and securing the eKYC functionality, this guide will provide you with the tools and knowledge needed to implement a robust and compliant verification system.
Integrate eKYC Processes With Blockchain Technology.
- Efficient User Verification: Streamline the process of verifying user identities with digital tools, reducing the time and effort required compared to traditional methods.
- Enhanced Security: Utilize blockchain’s robust security features to protect sensitive user data and ensure that verification processes are tamper-proof.
- Immutable Records: Store verification proofs on the blockchain for permanent, unchangeable records, providing a transparent and auditable trail of identity verification.
- Compliance Assurance: Meet regulatory requirements more effectively by leveraging digital records and automated processes that adhere to industry standards.
- Reduced Error Rates: Minimize the risk of human error and data discrepancies through automated verification and secure document storage.
Prerequisites
Before starting, ensure you have the following:
- Blockchain Environment: Set up a blockchain environment, such as Ethereum, Hyperledger, or a private blockchain.
- Node.js Installed: Ensure Node.js is installed on your machine.
- Smart Contract Development Tools: Tools like Truffle, Hardhat, or Remix for developing smart contracts.
- Basic Knowledge of Blockchain, Smart Contracts, and Node.js: Familiarity with these technologies will be helpful.
Step 1: Understanding eKYC Requirements
eKYC involves verifying the identity of users through digital means. The key components include:
- User Identity Verification: Collecting and verifying identity documents.
- Document Storage: Storing verified documents securely.
- Blockchain Integration: Storing verification proofs on the blockchain for immutability and transparency.
Step 2: Setting Up the Blockchain Environment
Start by setting up your blockchain environment. For this example, we'll use Ethereum and Truffle:
- Install Truffle and Ganache:
npm install -g truffle
npm install -g ganache-cli
-
Create a New Truffle Project:
truffle init eKYC-app
cd eKYC-app
This sets up a basic Truffle project structure.
-
Run Ganache:
Start a local Ethereum blockchain using Ganache:
ganache-cli
This local blockchain will simulate the Ethereum network for development purposes.
Step 3: Developing the Smart Contract
Create a smart contract to handle eKYC verification. In the contracts directory, create a new file KYC.sol:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
contract KYC {
struct User {
string name;
string documentHash;
bool isVerified;
}
mapping(address => User) public users;
address public admin;
modifier onlyAdmin() {
require(msg.sender == admin, "Only admin can perform this action");
_;
}
constructor() {
admin = msg.sender;
}
function addUser(string memory _name, string memory _documentHash) public {
users[msg.sender] = User(_name, _documentHash, false);
}
function verifyUser(address _userAddress) public onlyAdmin {
users[_userAddress].isVerified = true;
}
function isUserVerified(address _userAddress) public view returns (bool) {
return users[_userAddress].isVerified;
}
}
This smart contract does the following:
- User Structure: Defines a user structure with name, documentHash, and isVerified fields.
- Add User: Allows users to add their information and document hash.
- Verify User: Allows an admin to verify the user.
- Check Verification: Allows anyone to check if a user is verified.
Step 4: Deploying the Smart Contract
Next, deploy the smart contract to the local blockchain:
-
Create a Migration Script:
In the migrations directory, create a new file 2_deploy_kyc.js:
const KYC = artifacts.require("KYC");
module.exports = function (deployer) {
deployer.deploy(KYC);
};
-
Deploy the Contract:
Deploy the contract using Truffle:
truffle migrate --network development
This command deploys the KYC contract to the local blockchain.
Step 5: Integrating the eKYC Feature in a Node.js Backend
Now, let's set up a Node.js backend to interact with the smart contract and handle the eKYC process.
-
Set Up the Project:
In the project directory, initialize a Node.js project:
npm init -y
npm install web3 truffle-contract express body-parser
-
Create a Server File:
Create a new file server.js in the root directory:
const express = require('express');
const bodyParser = require('body-parser');
const Web3 = require('web3');
const contract = require('truffle-contract');
const KYCContract = require('./build/contracts/KYC.json');
const app = express();
app.use(bodyParser.json());
const web3 = new Web3(new Web3.providers.HttpProvider("http://localhost:8545"));
const KYC = contract(KYCContract);
KYC.setProvider(web3.currentProvider);
const adminAddress = "YOUR_ADMIN_ADDRESS"; // Replace with your admin address
app.post('/addUser', async (req, res) => {
const { name, documentHash, userAddress } = req.body;
try {
const instance = await KYC.deployed();
await instance.addUser(name, documentHash, { from: userAddress });
res.status(200).send("User added successfully");
} catch (error) {
res.status(500).send(error.toString());
}
});
app.post('/verifyUser', async (req, res) => {
const { userAddress } = req.body;
try {
const instance = await KYC.deployed();
await instance.verifyUser(userAddress, { from: adminAddress });
res.status(200).send("User verified successfully");
} catch (error) {
res.status(500).send(error.toString());
}
});
app.get('/isUserVerified/:userAddress', async (req, res) => {
const { userAddress } = req.params;
try {
const instance = await KYC.deployed();
const isVerified = await instance.isUserVerified(userAddress);
res.status(200).send({ isVerified });
} catch (error) {
res.status(500).send(error.toString());
}
});
app.listen(3000, () => {
console.log('Server running on port 3000');
});
This code does the following:
- Web3 Integration: Integrates Web3 to interact with the Ethereum blockchain.
- Express Server: Sets up an Express server to handle HTTP requests.
- User Management: Provides endpoints to add users, verify users, and check if a user is verified.
Step 6: Running and Testing the Application
To start your server and test the eKYC feature:
-
Start the Server:
node server.js
-
Test the Endpoints:
Use a tool like Postman to test the API endpoints:
-
Add User:
POST http://localhost:3000/addUser
Body: { "name": "John Doe", "documentHash": "Qm12345...", "userAddress": "0x123..." }
- Verify User:
POST http://localhost:3000/verifyUser
Body: { "userAddress": "0x123..." }
- Check Verification:
GET http://localhost:3000/isUserVerified/0x123...
Step 7: Areas to Be Careful About
- Data Privacy: Ensure that sensitive data such as user documents are stored securely and handled according to data protection regulations.
- Smart Contract Security: Write secure smart contracts to avoid vulnerabilities like reentrancy attacks or unauthorized access.
- Scalability: Consider the scalability of your eKYC solution, especially if you plan to deploy it on a public blockchain where transaction costs and speeds are variable.
Ready To Modernize Your Identity Verification Process?
Implement An eKyc Feature In Your Blockchain App And Ensure Secure, Efficient, And Compliant User Verification.
Conclusion
As digital platforms and regulations evolve, maintaining effective and compliant identity verification systems is crucial. By incorporating eKYC into your blockchain application, you’re adopting a forward-thinking approach that combines modern technology with rigorous security measures.
This guide has outlined the steps required to build an effective eKYC feature, including setting up your blockchain infrastructure, developing smart contracts, and integrating a backend system. The result is a sophisticated eKYC solution that ensures secure, transparent, and reliable user verification.
Moving forward, you may consider additional enhancements such as integrating with external KYC providers or implementing advanced security features to further fortify your system. By staying proactive and continually refining your approach, you’ll ensure your eKYC system remains robust and adaptable, meeting the evolving needs of both users and regulatory bodies.
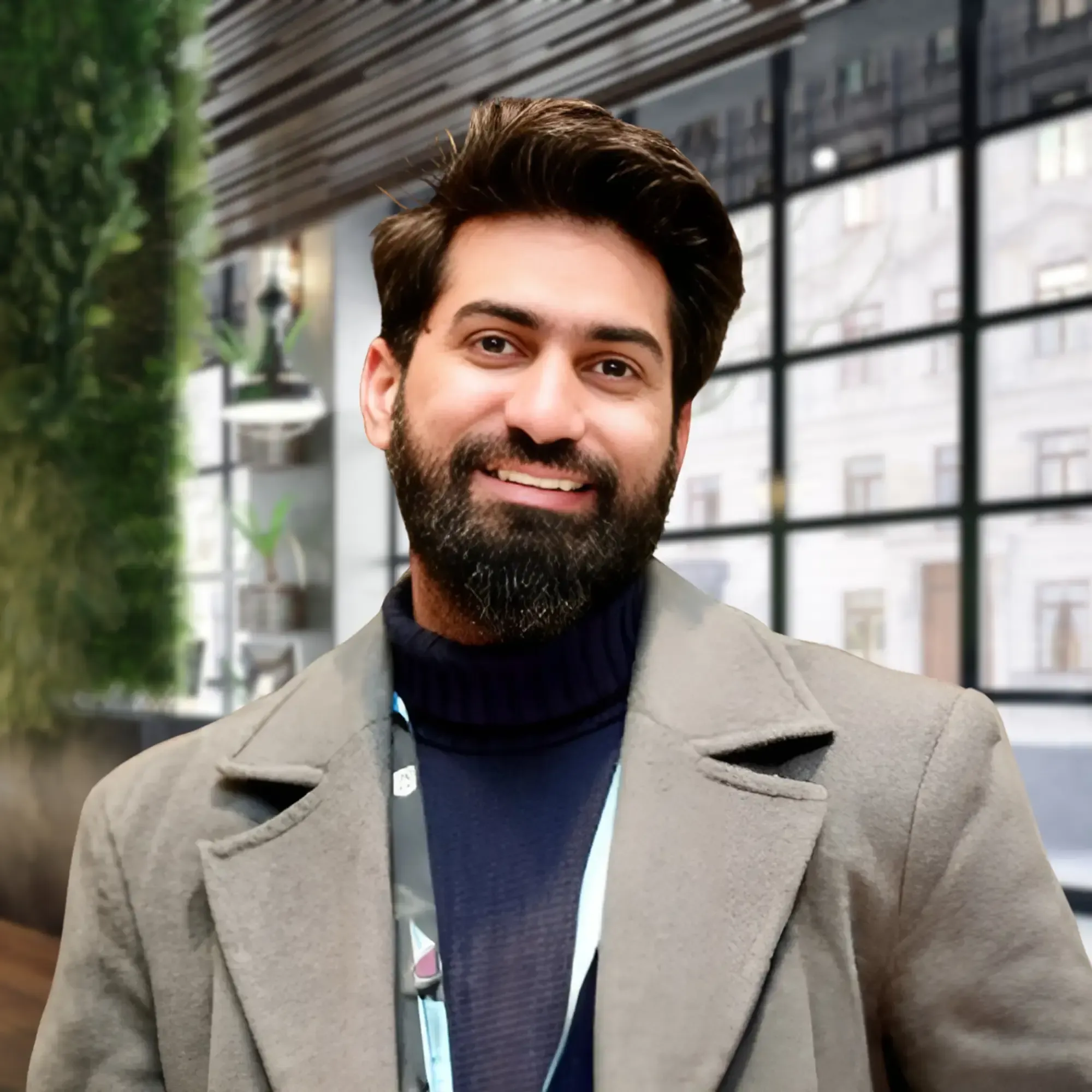
Written by :
Furqan Aziz
Furqan Aziz is CEO & Founder of InvoTeams. He is a tech enthusiast by heart with 10+ years of development experience & occasionally writes about technology here.